Scala.js
1.16.0
Harness the Scala and JavaScript ecosystems together.
Develop robust apps for browsers, Node.js, and serverless.
Correctness
Performance
Interoperability
Excellent editor support
With Scala.js, typos and type-errors are immediately caught and shown to you in your editor, without even needing to compile your code. Refactor any field or method with ease, with the confidence that if you mess it up the editor will tell you immediately. Stop flipping back and forth between your editor and MDN, because your editor will display what methods are available, what arguments they take, what they return, and even their documentation, right in-line with your code!
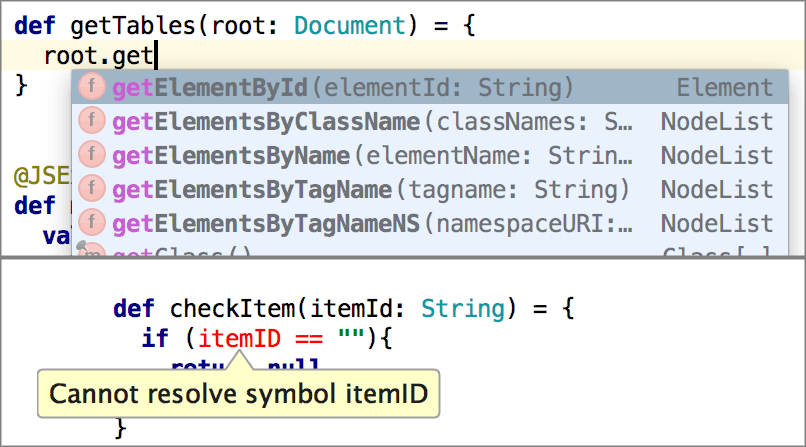
Go beyond JavaScript ES6, today
Hello World!
ECMAScript 6
console.log("Hello World!");
Scala.js
println("Hello World!")
Classes
ECMAScript 6
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
Scala.js
class Person(val firstName: String, val lastName: String) {
def fullName(): String =
s"$firstName $lastName"
}
Fat arrow functions
ECMAScript 6
const names = persons.map(p => p.firstName);
Scala.js
val names = persons.map(p => p.firstName)
// or an even shorter version
val names = persons.map(_.firstName)
Collections
ECMAScript 6
const personMap = new Map([
[10, new Person("Roger", "Moore")],
[20, new Person("James", "Bond")]
]);
const names = [];
for (const [key, person] of personMap) {
if (key > 15) {
names.push(`${key} = ${person.firstName}`);
}
}
Scala.js
val personMap = Map(
10 -> new Person("Roger", "Moore"),
20 -> new Person("James", "Bond")
)
val names = for {
(key, person) <- personMap
if key > 15
} yield s"$key = ${person.firstName}"
To find out more about what Scala.js looks like for JavaScript developers, check out the detailed comparisons. If you want to try it out yourself, check out the Tutorials!
Feature | JavaScript ES5 | JavaScript ES6 | TypeScript | Scala.js |
---|---|---|---|---|
Interoperability | ||||
Fully EcmaScript5 compatible | ||||
No compilation required | ||||
Use existing JS libraries | ||||
Language features | ||||
Classes | ||||
Modules | ||||
Support for types | ||||
Strong type system | ||||
Extensive standard libraries | ||||
Optimizing compiler | ||||
Macros, to extend the language | ||||
IDE support | ||||
Catch most errors in IDE | ||||
Easy and reliable refactoring | ||||
Reliable code completion |